“`html
MongoDB is a NoSQL database that aids in various tasks to build robust applications. When managing large databases, locating text within extensive datasets can be difficult and unproductive. This is where MongoDB’s text search feature comes into play, converting queries into text. The text search functionality in MongoDB is tailored for relevance-focused matching, allowing users to swiftly uncover the most pertinent results, making it perfect for search bars, product catalogs, and document search capabilities. In this article, we will explore the text search, its features, and its performance.
Table of Contents:
- What is MongoDB Text Search?
- Why Is Text Search Important in MongoDB?
- How to Configure MongoDB Text Search?
- Considerations for Performance
- Recommended Practices
- Wrap-Up
What is MongoDB Text Search?
Text search is a feature utilized to locate strings within a text-based document. This function assists in retrieving all related documents that contain the specified string, even if it does not fully match all provided strings. It yields results if just one word in the string corresponds with the document.
For instance, if we are searching for the string “Book,”, the text search will retrieve all instances related to Book, including results such as “The Book Thief,” since The Book Thief contains the word ‘book’, thus being recognized as a match.
Why Is Text Search Important in MongoDB?
Conventional querying methods are often ineffective and can be time-intensive, particularly when dealing with large datasets. By employing text search, if an individual wishes to search for “Data Science,” the text search ignores case sensitivity and surrounding words. If any term is linked to data science, the search will identify it and present it as a result. It can also be useful when searching for comments across multiple fields concerning a specific topic; the text search will search and return results. It offers greater flexibility compared to regex functions or precise match-finding techniques.
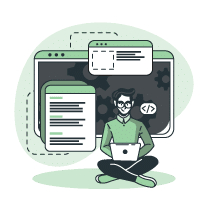
How to Configure MongoDB Text Search?
Prior to executing a text search in a document, you must establish a text index for the fields in the document you intend to search. Setting up a text index is the initial step to utilizing text search within documents.
What is a text index?
A text index in MongoDB is utilized to enhance the querying process. It retains references to keywords within string fields, facilitating effective text searches. Without a text index, MongoDB needs to scan the entire document to find specific text, leading to decreased query performance. A text index enables MongoDB to search efficiently for terms within string content. For example, if a document contains the term ‘books’, a search for ‘book’ could still return it based on keyword relevance. You can establish a text index on one or more fields depending on your search needs.
Syntax:
db.collection.createIndex({ field1: "text", field2: "text" });
Example:
db.blogs.createIndex({ title: "text", FAQ: "text" });
Explanation: This command will create an index on the title and FAQ fields in the document or blog collection.
Drawbacks of Text Index
- Each collection can contain only one text index, but that index can encompass multiple fields.
- Introducing the text string may increase the size of the database, compromising the write performance of the database.
- Only strings or arrays of string values in indexed fields are taken into account during text searches.
- Text indexes do not support sorting on fields beyond the relevance score (textScore) without employing a compound index.
Searching for a Single Text in a Collection
After the text index has been established, you can execute a text search using the $text operator.
db = client['mydatabase']
Intellipaat = db['Intellipaat']
Intellipaat.insert_many([
{
"title": "Wings of Fire",
"description": "This is a book about Dr. A.P.J. Kalam."
},
{
"title": "The Book Thief",
"description": "A tale of a young girl's affection for books."
},
{
"title": "Introduction to Finance",
"description": "An article published by Intellipaat."
}
])
Intellipaat.create_index([('title', 'text'), ('description', 'text')])
query = { "$text": { "$search": ``````html "Book" } }
print("Documents corresponding to 'Book':n")
for document in Intellipaat.find(query):
print(document)
Output:

Explanation: The results of all text searches will be enclosed within the { }, and each will possess its unique index termed as _id reflecting the text index.
To Query an Array of Strings Within a Collection
db = client['mydatabase']
Intellipaat = db['Intellipaat']
Intellipaat.drop()
Intellipaat.insert_many([
{
"title": "Full-Text Search in Databases",
"content": "Databases employ text indexes to execute search operations."
},
{
"title": "Text and Search Fundamentals",
"content": "Core principles of text search capabilities."
},
{
"title": "Learn to Cook Easily",
"content": "Simple method of Learning Cooking."
}
])
Intellipaat.create_index([('title', 'text'), ('content', 'text')])
query = {
"$text": { "$search": ""text search"" }
}
results = Intellipaat.find(query)
for document in results:
print(document)
Output:

Explanation: Here, the results appeared as Text and Search Fundamentals and Full-Text Search in Database, as those documents include the exact phrase ‘text search‘.
Excluding or Omitting Strings During Text Search
Occasionally, when you wish to omit certain words, it’s comparable to specifying one term to retrieve but not the other.
For instance, “Python -SQL” will seek the term Python but eliminate the term SQL.
db = client['mydatabase']
Intellipaat = db['Intellipaat']
Intellipaat.insert_many([
{ "title": "Learn Python", "content": "Learn Python Effortlessly." },
{ "title": "History of SQL and Python", "content": "Chronology and integration of SQL and Python." },
{ "title": "Python for Data Science", "content": "Python is employed in machine learning." },
{ "title": "Basics of SQL", "content": "Understand database management." },
])
Intellipaat.create_index([('title', 'text'), ('content', 'text')])
query = { "$text": { "$search": "Python -SQL" } }
results = Intellipaat.find(query)
for Intellipaat in results:
print(Intellipaat)
Output:

Explanation: In this instance, the $search identified the output for Python and discarded any output that included SQL.
Querying Text With Operators
These query operators in NoSQL are conditions used to retrieve the text string based on specific criteria, similar to the “WHERE” or “IN” operators in SQL. Some operators include $gt, $lt, and $in, which serve as filters to search the text string with conditions.
Example:
from datetime import datetime
from pymongo import MongoClient
db = client['mydatabase']
articles = db['articles']
articles.drop()
articles.insert_many([
{
"title": "Introduction to MongoDB",
"content": "MongoDB represents a NoSQL database.",
"publishedDate": datetime(2023, 7, 19)
},
{
"title": "Advanced MongoDB Queries",
"content": "Discover queries in MongoDB.",
"publishedDate": datetime(2023, 6, 24)
},
{
"title": "Basics of SQL Databases",
"content": "Understand SQL databases.",
"publishedDate": datetime(2022, 2, 27)
},
{
"title": "MongoDB or SQL",
"content": "Distinction between MongoDB and SQL databases.",
"publishedDate": datetime(2022, 1, 3)
},
{
"title": "Learn Web Development",
"content": "Comprehensive approach to web development.",
"publishedDate": datetime(2023, 2, 1)
}
])
articles.create_index([('title', 'text'), ('content', 'text')])
query = {
"$text": {"$search": "MongoDB"},
"publishedDate": {"$gt": datetime(2023, 1, 1)}
}
results = articles.find(query)
for document in results:
print(document)
Output:

Explanation: Here, the $gt yielded only the document that has a publication date in 2023.
Performance Considerations
- The dimension of every index in the database necessitates more storage, potentially impacting performance and capacity.
- Text searching is typically slower compared to exact matching queries, as text searches will return all relevant results from the database.
- Text indexes perform less effectively with high-cardinality fields (numerous unique words). Refrain from indexing fields with highly variable lexicon unless necessary.
- The default text index in MongoDB disregards common stop words (such as “the”, “and”). It also utilizes stemming, which may match variations (e.g., “run”, “running”).
Best Practices
- Always determine the specific fields you intend to search for text; this can ameliorate performance.
- Strive to minimize the number of search results returned; this can help reduce memory consumption.
- Text searches are consistently case-insensitive.
- MongoDB’s text search typically disregards common terms during querying, such as “the, is, or, from,
“““html
that, etc.
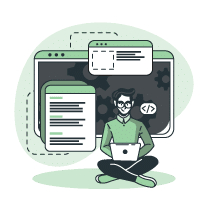
Final Thoughts
Text Search is a vital utility in MongoDB that allows you to look up text strings without requiring the installation of search engines or integration utilities. By acquiring skills in text search, you can strategically apply search parameters to sift through results according to your defined criteria. By grasping the foundational structure and functionality, you can effectively conduct searches for any text string with ease and efficiency. This capability is particularly advantageous in apps such as blogs, e-commerce sites, and content management systems where text-based inquiries are common.
To delve deeper into SQL functions, visit this MongoDB and also peruse MongoDB Interview Questions crafted by industry specialists.
MongoDB Text Search – Frequently Asked Questions
To search text within MongoDB, generate a text index on the relevant fields and employ the $text operator along with the $search keyword to locate matching documents.
Utilize db.collection.createIndex({ field: “text” }) to create a text index in MongoDB.
Use the query { $text: { $search: “your search string” } } to identify matching documents in MongoDB.
Text-based search retrieves documents based on coinciding keywords or phrases found in text fields.
MongoDB performs well for basic full-text search, but for more complex requirements, tools such as Elasticsearch are preferable.
The post MongoDB Text Search appeared first on Intellipaat Blog.
“`