When working with HTML, you might encounter the terms “properties” and “attributes”. In the context of the DOM (Document Object Model), they fulfill distinct functions, despite their apparent similarities. This article will explore their differences, behaviors, and usage in JavaScript through examples for enhanced comprehension.
Table of Contents:
- What are Attributes?
- What are Properties?
- Differences Between Attributes and Properties
- When to Use Attributes vs. Properties
- Conclusion
What are Attributes?
Attributes are the values you assign to an HTML element within your code. They allow you to define the initial state of an element, and you can also directly place these values in your HTML file.
Example:
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<meta name="viewport" content="width=device-width, initial-scale=1.0">
<title>Disabled Input Example</title>
</head>
<body>
<h2>Disabled Input Field Example</h2>
<form>
<label for="username">Username:</label>
<input type="text" id="username" value="Intellipaat.aa" disabled>
<br><br>
<label for="email">Email:</label>
<input type="email" id="email" placeholder="Enter your email">
<br><br>
<button type="submit">Submit</button>
</form>
</body>
</html>
Output:
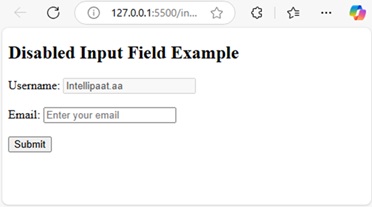
Explanation: In this snippet, the type, id, value, and disabled are all considered attributes of the <input> element. These attributes dictate how the element behaves and appears upon the initial loading of the page.
What are Properties?
Properties are components of the DOM, which encapsulate the elements in your code. They function akin to characteristics of a JavaScript object connected to an element. You can modify these properties using JavaScript without altering the underlying HTML structure.
Example:
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<meta name="viewport" content="width=device-width, initial-scale=1.0">
<title>Input Value Example</title>
</head>
<body>
<h2>Retrieve and Modify Input Value Example</h2>
<label for="username">Username:</label>
<input type="text" id="username" value="Intellipaat.aa" disabled>
<br><br>
<button onclick="updateInput()">Enable & Modify Value</button>
<script>
function updateInput() {
let inputElement = document.getElementById("username");
console.log(inputElement.value);
inputElement.disabled = false;
inputElement.value = "XYZ";
console.log(inputElement.value);
}
</script>
</body>
</html>
Output:
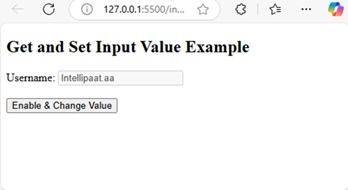
Explanation: The value attributed to <input> is a property within the DOM. It reflects the present content of the input field, and you can dynamically change it using JavaScript.
Differences Between Attributes and Properties
Feature | Attributes | Properties |
Definition | Defined in the HTML markup. | Defined in the DOM structure. |
Access method | Utilize getAttribute() and setAttribute() |
Accessed directly as properties of the DOM object. |
Example | setAttribute(“value”, “Intellipaat”) |
Element.value = “Intellipaat” |
Key Differences in Behavior
Attributes Specify the Initial State, While Properties Indicate the Current State:
Attributes can assign values to elements at the time of the page’s initial loading, while properties represent the ongoing value of the element as it changes.
console.log(inputElement.getAttribute("value"));
console.log(inputElement.value);
After altering inputElement.value
, it does not modify inputElement.getAttribute(“value”). Attributes remain constant as they were originally established in HTML, regardless of any dynamic changes to properties.
Boolean Attributes vs. Boolean Properties:
Attributes such as checked, disabled, and read-only are referred to as boolean attributes in HTML. When accessing them as properties in JavaScript, they exhibit different behaviors compared to their HTML functionality.
<input type="checkbox" id="agree" checked>
let checkbox = document.getElementById("agree");
console.log(checkbox.getAttribute("checked"));
“`javascript
console.log(checkbox.checked);
checkbox.checked = false;
console.log(checkbox.getAttribute(“checked”));
console.log(checkbox.checked);
Utilizing getAttribute(“checked”) provides an empty string if the attribute is present in the HTML. In contrast, the checked property in JavaScript yields either true or false, based on the current status of the element in the DOM.
When to Utilize Attributes vs. Properties
- Attributes can be employed to define values indicated by the HTML, such as data-* attributes.
- Properties are utilized to interact with the DOM and implement JavaScript functions to modify the elements.
Examples:
Utilizing data-* Attributes
<button id="btn" data-action="submit">Click Me</button>
Altering Properties:
button.textContent = "Submit Form";
console.log(button.getAttribute("textContent"));
Summary
Attributes are suitable for initial configuration and establishing values, while properties are employed to ascertain the current condition of the DOM and engage with elements for alterations. Understanding their distinction is crucial for effectively managing these components on your webpage.
What distinguishes Properties from Attributes in HTML? – FAQs
Attributes can be employed to set values directly within the HTML markup.
This can be used to retrieve the current status of the element and alter it using JavaScript.
Attributes establish the initial condition (e.g., value in <input>) while properties track real-time modifications.
No, altering a property (e.g., element.value = “newValue”) does not update the related attribute (getAttribute(“value”)).
Boolean attributes such as checked, disabled, and readonly operate differently in JavaScript compared to HTML. When present as attributes in HTML, they are represented as empty strings.
The article What is the Difference Between Properties and Attributes in HTML? initially appeared on Intellipaat Blog.
“`